目次
非同期処理とは
非同期処理とは実行中、別のタスクを行うことができる方式のことをいいます
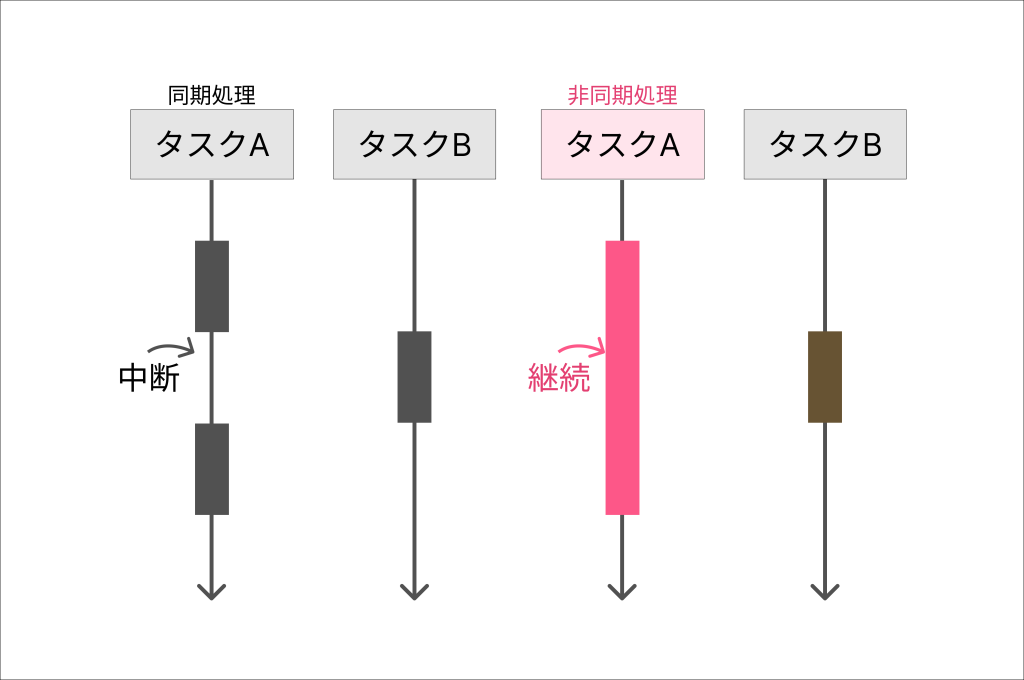
非同期処理は制御が難しい
重い処理もユーザを待たせずほかの操作がサクサク可能となる(グーグルマップ等)メリットはありますが、デメリットもあります。
実行完了していない状態で、エラーがおきることがあります。
(原因の例) 「実行完了までデータが存在しない」という状態
→Promiseによって実行完了を待つことができます
Promiseオブジェクト
Promiseオブジェクトは3つの状態をもちます
「Promiseの状態」
- pending(保留): 初期状態。まだ非同期処理は終わっていない(成功も失敗もしていない)
- fulfilled(成功): 非同期処理が正常に終了した
- rejected(拒否): 非同期処理が失敗した
Promiseを使った非同期処理の実装
1)失敗例:「fetch」によるGitHubのID取得を「待たずに」処理が実行
JavaScriptのfetch()メソッド
「非同期通信でリクエストを発行、そのレスポンスを取得」することができる関数
// 非同期処理をおこなう関数を宣言
const getGitUsername = () => {
const url = 'https://api.github.com/users/ida240609'
// GitHub APIをFetchメソッドで実行
fetch(url).then(res => res.json())
.then(json => {
console.log('これは非同期処理成功時のメッセージです')
return json.login
}).catch(error => {
console.error('これは非同期処理失敗時のメッセージです。', error)
return null
})
};
const message = 'GitのユーザーIDは'
const username = getGitUsername()
console.log(message + username)
console↓
GitのユーザーIDはundefined
これは非同期処理成功時のメッセージです
2)Promise:fetchによるGitHubのID取得「完了後」に処理が実行
// import fetch from 'node-fetch';
// 非同期処理をおこなう関数を宣言
const getGitUsernamePromise = () => {
return new Promise((resolve, reject) => {
const url = 'https://api.github.com/users/ida240609'
// GitHub APIをFetchメソッドで実行
fetch(url).then(res => res.json())
.then(json => {
console.log('これは非同期処理成功時のメッセージです')
return resolve(json.login)
}).catch(error => {
console.error('これは非同期処理失敗時のメッセージです。', error)
return reject(null)
})
})
};
const message = 'GitのユーザーIDは'
getGitUsernamePromise().then(username => {
console.log(message + username)
});
console↓
これは非同期処理成功時のメッセージです(Promise)
GitのユーザーIDはida240609
2)async/await:fetchによるGitHubのID取得「完了後」に処理が実行
// 非同期処理をおこなう関数を宣言
const getGitUsernameAsyncAwait = async () => {
const message = 'GitのユーザーIDは';
const url = 'https://api.github.com/users/deatiger'
const json = await fetch(url)
.then(res => {
console.log('これは非同期処理成功時のメッセージです(AsyncAwait)')
return res.json()
}).catch(error => {
console.error('これは非同期処理失敗時のメッセージです(AsyncAwait)', error)
return null
});
const username = json.login;
console.log(message + username)
}
getGitUsernameAsyncAwait()
console↓
これは非同期処理成功時のメッセージです(AsyncAwait)
GitのユーザーIDはida240609
async/await
を使うには、Promiseが必要 多くの現代的なAPIは内部で自動的にPromiseを返す だから普段はPromiseを意識せずにasync/await
が使える